What is Rendering?
Rendering is the process of transforming the component code you write into UI that users can see and interact with
In Next.js, the tricky part to building a performant application is figuring out when and where this transformation should happen.
CSR, SSR and RSCs?
Rendering in React โ Rendering in Next.js
How rendering works in React - CSR
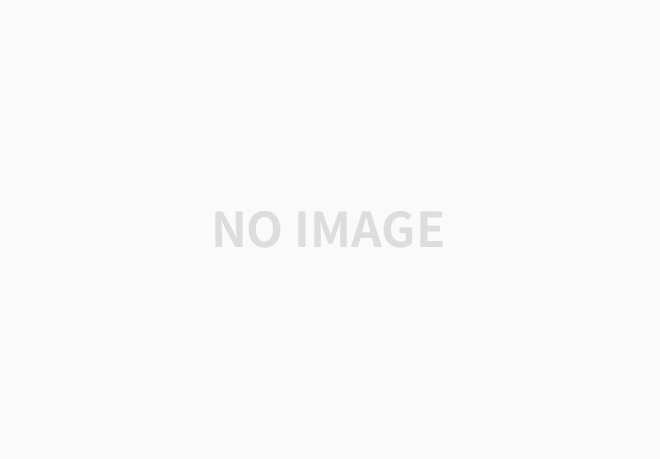
Client Side Rendering
This whole approach - where your browser transforms React components into what you see on screen - that is what we call client-side rendering
Drawbacks of CSR
- SEO
- When search engines crawl your site, they are mainly looking at HTML content. But with CSR, your initial HTML is basically just an empty div - not great for search engines trying to figure out what your page is about
- Performance
- Your browser(the client) has to do everything: fetch data, build the UI, make everything interactive. thatโs a lot of work!
- Users often end up staring at a blank screen or loading spinner while all this happens.
Server Side Rendering
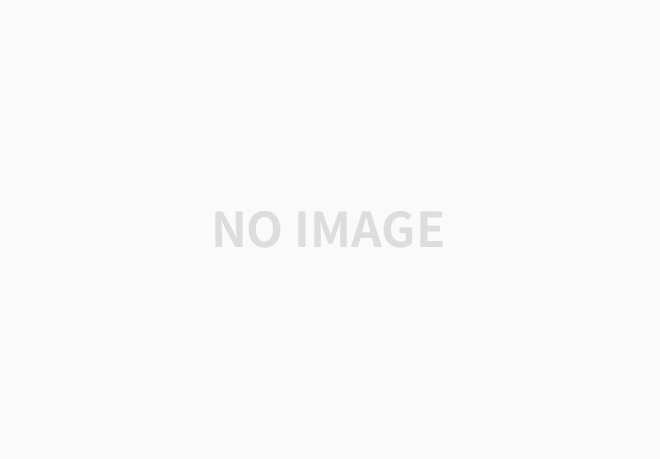
Server-side solutions
Search engines can now easily index the server-rendered content, solving our SEO problem.
Users see actual HTML content right away instead of staring at a blank screen or loading spinner.
- Static Site Generation (SSG)
- SSG happens during build time when you deploy your application to the server.
- This results in pages that are already rendered and ready to serve. Itโs perfect for content that stays relatively stable, like blog posts.
- Server-Side Rendering (SSR)
- SSR renders pages on-demand when users request them. Itโs ideal for personalized content like social media feeds where the HTML changes based on whoโs logged in.
Hydration
During hydration, React takes control in the browser and reconstructs the component tree in memory, using the server-rendered HTML as a blueprint.
This involves initializing application state, adding click and mouseover handler, and setting up all the dynamic features needed for a full interactive user experience.
Drawbacks of SSR - All or nothing
- You have to fetch everything before you can show anything. We canโt start rendering HTML until all data is fetched on the server.
- You have to fetch everything before you can hydrate anything. We need to wait for all JS to load on the client before hydration can begin.
- You have to hydrate everything before you can interact with anything. Every component needs to be hydrated before any of them become interactive.
This becomes really inefficient when some parts of your app are slower than others, as is often the case in real-world apps.
Suspense SSR architecture
use the component to unlock two major SSR features:
- HTML streaming on the server
- HTML streaming solves our first problem: You donโt have to fetch everything before you can show anything
- If a particular section is slow and could potentially delay the initial HTML, NP!
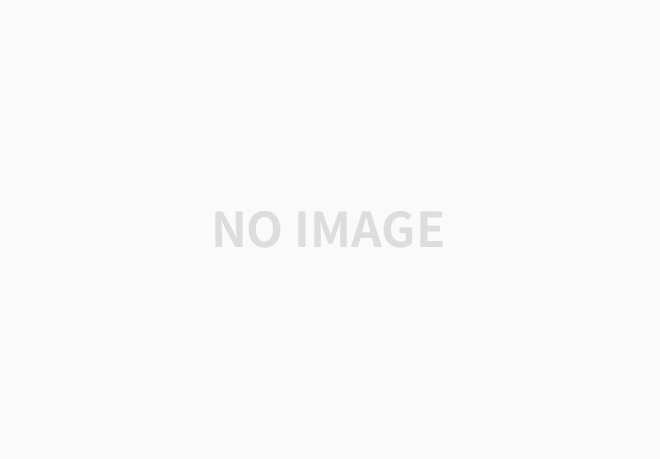
- Selective hydration on the client
- By wrapping your main section in a
<Suspense>
component, youโre not just enablign streaming but also telling React itโs okay to hydrate other parts of the page before everythingโs ready.
- By wrapping your main section in a
The Evolution of React
CSR โ SSR โ Suspense for SSR
Suspense for SSR brought us closer to a seamless rendering experience.
But, we still have challenges
- Large bundle sizes causing excessive downloads for users
- Unnecessary hydration delaying interactivity
- Heavy client-side processing leading to poorer performance
React Server Components (RSC)
RSC represent a new architecture designed by React team.
This approach leverages the strengths of both server and client environments to optimize efficiency, load times, and interactivity
The architecture introduces a dual-component model
- CSR
- SSR
This distinction is based not on the componentโs functionality but rather on their execution environment and the specific systems they are designed to interact with.
Server Components handle data fetching and static rendering, while Client Components take care of rendering the interactive elements.
RSC + Next.js
Every component in a Next.js app defaults to being a server component
Running components on the server brings several advantages: aero-bundle size, direct access to server-side resources, improved security, and better SEO.
But, Server components canโt interact with browser APIs or handle user interactions.
// about/page.tsx import { useState } from "react"; export default function About() { const [name, setName] = useState(""); return <h1>about page!</h1>; }
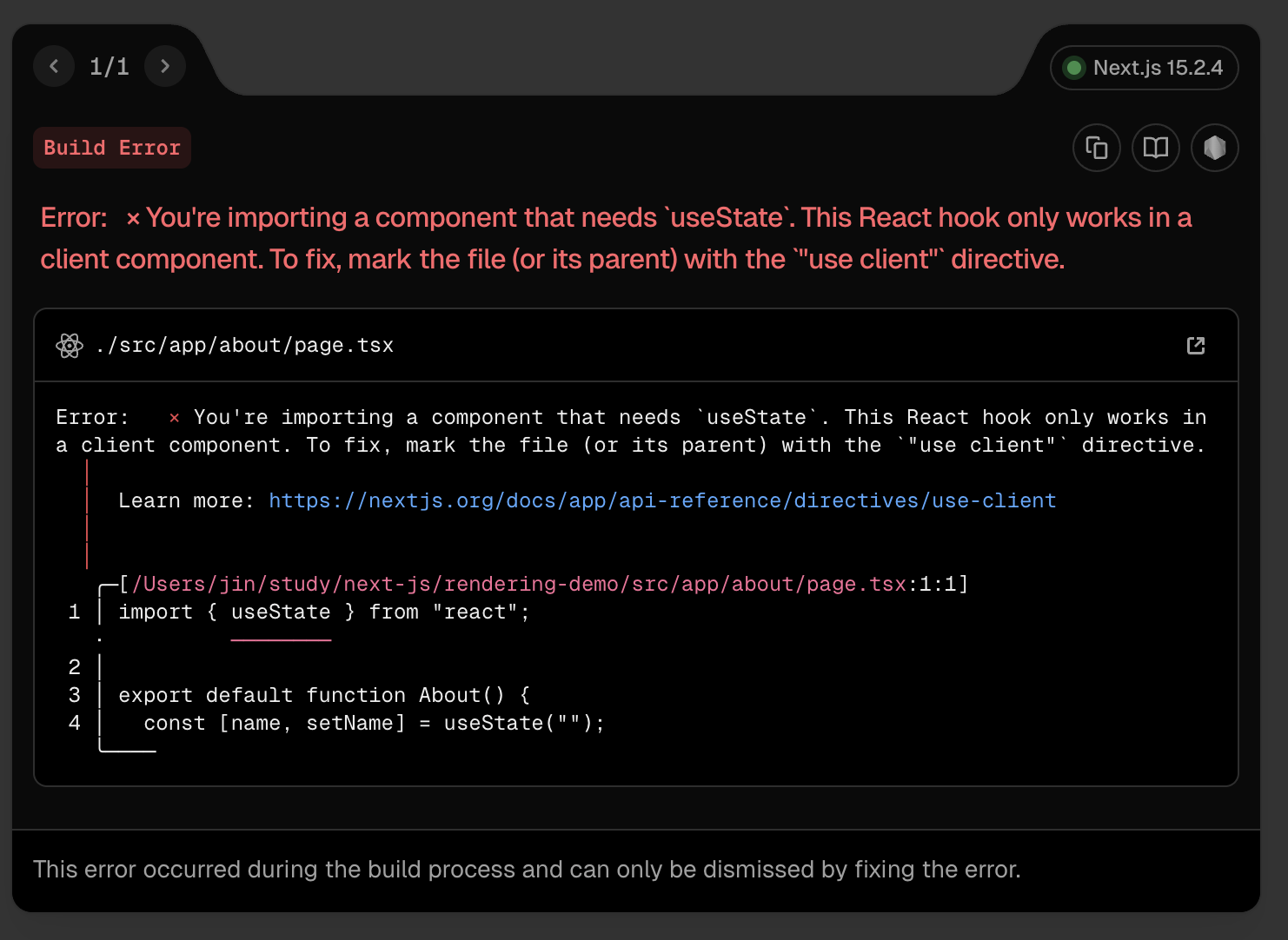
- To create components, add the โuse clientโ directive at the top of the file.
- Server components are rendered exclusively on the server.
- Client components are rendered once on the server and then on the client.
Reference
'๐จโ๐ป Programming > Next.js' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[Next.js] Next.js ์๊ฐ (0) | 2025.03.25 |
---|